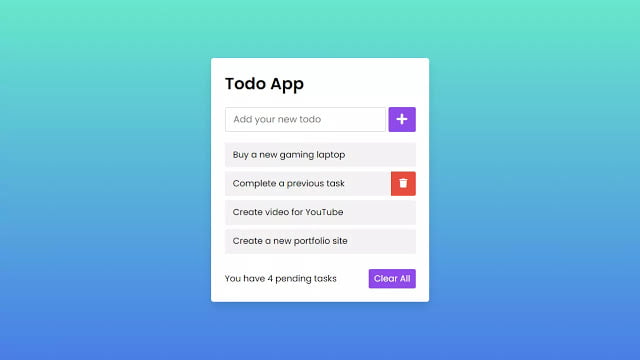
So today I came with another blog where I create Todo App and the lists or tasks that you add won’t be removed when you refresh the page and in this todo app there are also features of pending tasks number and you can also delete all tasks that you have added on single click.
As you may know, A to-do list is a list of tasks you need to complete or things you want to do and in our design [Todo List App], there is a content box that holds only the input field with some buttons and text. When you entered some characters and click on the plus (+) button, the list will be added to your tasks list and the number of the pending tasks also be updated.
You can also delete each list by clicking on the trash icon, and remember this trash icon only appear on hover on the particular list and delete all tasks with a single click. If you want to see this to-do list app and how it is created then you can watch a full video tutorial on this program [Todo List App in JavaScript].
Video Tutorial of Todo List App in JavaScript
In the video, you have seen the to-do list app and how it is created using HTML CSS & JavaScript. I hope you’ve understood the codes behind creating this program. It may be difficult to understand the JavaScript codes of this program but don’t worry once you download the code files of this program and try with yourself, you’ll easily understand.
You may wonder about how these to-do app tasks remain even after refreshing the webpage right? Well, at first, the tasks you’ve added will store in your browser’s local storage and then these lists are retrieved into an HTML file in the form <li> tag using JavaScript.
You may feel difficult to understand what I’m saying right? If yes then you’ve to watch the video again and again because in the video I have written the comment of each JavaScript line and tried my best to understand the codes for you.
You might like this:
Todo List App in JavaScript [Source Codes]
To create this program [Todo List App]. First, you need to create three files, HTML File, CSS File, and JavaScript File. After creating these files just paste the following codes into your files. You can also download the source code files of the to-do list app from the given download button.
First, create an HTML file with the name of index.html and paste the given codes in your HTML file. Remember, you’ve to create a file with .html extension.
<!DOCTYPE html> <!-- Created By CodingNepal - www.codingnepalweb.com --> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Todo App JavaScript | CodingNepal</title> <link rel="stylesheet" href="style.css"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css"/> </head> <body> <div class="wrapper"> <header>Todo App</header> <div class="inputField"> <input type="text" placeholder="Add your new todo"> <button><i class="fas fa-plus"></i></button> </div> <ul class="todoList"> <!-- data are comes from local storage --> </ul> <div class="footer"> <span>You have <span class="pendingTasks"></span> pending tasks</span> <button>Clear All</button> </div> </div> <script src="script.js"></script> </body> </html>
Second, create a CSS file with the name of style.css and paste the given codes in your CSS file. Remember, you’ve to create a file with .css extension.
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap'); *{ margin: 0; padding: 0; box-sizing: border-box; font-family: 'Poppins', sans-serif; } ::selection{ color: #ffff; background: rgb(142, 73, 232); } body{ width: 100%; height: 100vh; /* overflow: hidden; */ padding: 10px; background: linear-gradient(to bottom, #68EACC 0%, #497BE8 100%); } .wrapper{ background: #fff; max-width: 400px; width: 100%; margin: 120px auto; padding: 25px; border-radius: 5px; box-shadow: 0px 10px 15px rgba(0,0,0,0.1); } .wrapper header{ font-size: 30px; font-weight: 600; } .wrapper .inputField{ margin: 20px 0; width: 100%; display: flex; height: 45px; } .inputField input{ width: 85%; height: 100%; outline: none; border-radius: 3px; border: 1px solid #ccc; font-size: 17px; padding-left: 15px; transition: all 0.3s ease; } .inputField input:focus{ border-color: #8E49E8; } .inputField button{ width: 50px; height: 100%; border: none; color: #fff; margin-left: 5px; font-size: 21px; outline: none; background: #8E49E8; cursor: pointer; border-radius: 3px; opacity: 0.6; pointer-events: none; transition: all 0.3s ease; } .inputField button:hover, .footer button:hover{ background: #721ce3; } .inputField button.active{ opacity: 1; pointer-events: auto; } .wrapper .todoList{ max-height: 250px; overflow-y: auto; } .todoList li{ position: relative; list-style: none; margin-bottom: 8px; background: #f2f2f2; border-radius: 3px; padding: 10px 15px; cursor: default; overflow: hidden; word-wrap: break-word; } .todoList li .icon{ position: absolute; right: -45px; top: 50%; transform: translateY(-50%); background: #e74c3c; width: 45px; text-align: center; color: #fff; padding: 10px 15px; border-radius: 0 3px 3px 0; cursor: pointer; transition: all 0.2s ease; } .todoList li:hover .icon{ right: 0px; } .wrapper .footer{ display: flex; width: 100%; margin-top: 20px; align-items: center; justify-content: space-between; } .footer button{ padding: 6px 10px; border-radius: 3px; border: none; outline: none; color: #fff; font-weight: 400; font-size: 16px; margin-left: 5px; background: #8E49E8; cursor: pointer; user-select: none; opacity: 0.6; pointer-events: none; transition: all 0.3s ease; } .footer button.active{ opacity: 1; pointer-events: auto; }
Last, create a JavaScript file with the name of script.js and paste the given codes in your JavaScript file. Remember, you’ve to create a file with .js extension.
// getting all required elements const inputBox = document.querySelector(".inputField input"); const addBtn = document.querySelector(".inputField button"); const todoList = document.querySelector(".todoList"); const deleteAllBtn = document.querySelector(".footer button"); // onkeyup event inputBox.onkeyup = ()=>{ let userEnteredValue = inputBox.value; //getting user entered value if(userEnteredValue.trim() != 0){ //if the user value isn't only spaces addBtn.classList.add("active"); //active the add button }else{ addBtn.classList.remove("active"); //unactive the add button } } showTasks(); //calling showTask function addBtn.onclick = ()=>{ //when user click on plus icon button let userEnteredValue = inputBox.value; //getting input field value let getLocalStorageData = localStorage.getItem("New Todo"); //getting localstorage if(getLocalStorageData == null){ //if localstorage has no data listArray = []; //create a blank array }else{ listArray = JSON.parse(getLocalStorageData); //transforming json string into a js object } listArray.push(userEnteredValue); //pushing or adding new value in array localStorage.setItem("New Todo", JSON.stringify(listArray)); //transforming js object into a json string showTasks(); //calling showTask function addBtn.classList.remove("active"); //unactive the add button once the task added } function showTasks(){ let getLocalStorageData = localStorage.getItem("New Todo"); if(getLocalStorageData == null){ listArray = []; }else{ listArray = JSON.parse(getLocalStorageData); } const pendingTasksNumb = document.querySelector(".pendingTasks"); pendingTasksNumb.textContent = listArray.length; //passing the array length in pendingtask if(listArray.length > 0){ //if array length is greater than 0 deleteAllBtn.classList.add("active"); //active the delete button }else{ deleteAllBtn.classList.remove("active"); //unactive the delete button } let newLiTag = ""; listArray.forEach((element, index) => { newLiTag += `<li>${element}<span class="icon" onclick="deleteTask(${index})"><i class="fas fa-trash"></i></span></li>`; }); todoList.innerHTML = newLiTag; //adding new li tag inside ul tag inputBox.value = ""; //once task added leave the input field blank } // delete task function function deleteTask(index){ let getLocalStorageData = localStorage.getItem("New Todo"); listArray = JSON.parse(getLocalStorageData); listArray.splice(index, 1); //delete or remove the li localStorage.setItem("New Todo", JSON.stringify(listArray)); showTasks(); //call the showTasks function } // delete all tasks function deleteAllBtn.onclick = ()=>{ listArray = []; //empty the array localStorage.setItem("New Todo", JSON.stringify(listArray)); //set the item in localstorage showTasks(); //call the showTasks function }
That’s all, now you’ve successfully created a Todo List App using HTML CSS & JavaScript. If your code doesn’t work or you’ve faced any error/problem then please download the source code files from the given download button. It’s free and a .zip file will be downloaded then you’ve to extract it.
Parse error on line 1:
// getting all requi
^
Expecting ‘STRING’, ‘NUMBER’, ‘NULL’, ‘TRUE’, ‘FALSE’, ‘{‘, ‘[‘, got ‘undefined’
I keep getting this message once I try to validate the js so what does it mean and how to fix it?
Hi, is it possibile to make this able to accept links and let them active once put in the list?By the way great code and thanks
Hello, question: I keep getting an “Uncaught TypeError: inputBox is null” with this code. I have no idea what’s causing it, but it happens across all my browsers when I test it. It’s not just my copying it bad – even copy paste doesn’t help! I’ve tried figuring out what could be wrong but I don’t know… any suggestions or ideas?
You’ve may have done mistakes in the code. So, download the codes file from the download button.
Create A Todo List App in HTML CSS & JavaScript | Todo App in JavaScript
(please bro hariup code 2022 feb20 video )
Here is the code bro – https://www.codingnepalweb.com/create-todo-list-app-html-javascript/
Hey any tips on how I can add an update functionality? Like I press a todo I get the option to update it. Moreover, Can you make a tutorial on how to implement Cloudfare KV instead of local storage.
What keywords should I search for
please help me coding nepal
I’m really sorry. I don’t know about it but you can google it for quick and accurate answers.
Ok
Can you tell me briefly about the production principle of the recycle bin? Then I tried to make it myself please help me coding nepal
Thank you for sharing. This is amazing. The code runs just well on my personal computer.
I have a “todo app” project to submit to the Research and Development Department at where I work, and I have decided to use yours, tweak it to make it feel more personal and pitch it.
The odds are in my favor and I am giving you the credit for this great code. I would land my first paying developer job because of your good efforts in sharing this.
Thank you very much CodingNepal. Cheers 🙂
Happy to hear that… Keep visiting <3
Can you simply explain to me how to make a recycle bin, and then I try to make it myself
Can you tell me briefly about the production principle of the recycle bin? Then I tried to make it myself
hey ,i have a question ,what does “link rel=”stylesheet” href=”https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css”/” do ?
ps: thank you for the your work
This is a CDN (Content Delivery Network) link of FontAwesome and it’s used for icons.
Nice
How can I make a simple online word processor with HTML, CSS and JavaScript.
Please
bro , your code is not working for long strings ,meaning if i put 7,8 words then there is no option for deleting that list
Thanks for informing me. It has been fixed and, now you can download or copy the updated codes.
wow
hi its awesome but iw as wondering, how would you be able to create 3 inputs and 3 outputs. for example instead of “ad your new todo” maybe a few more like “favorate meal, time you want to eat it, date” and out put these three. where would i put that in the js, css, and html so it outputs all 3″
i will try this with firebase from google or aws from amazon and then creating a pwa-app then you can use this on the phone too
Which templete/theme you are using in you website
I would love to save the todo list on my server, so I can access it from mobile or pc anytime. How can I achieve that?
Bro it's a default comment system of Blogger.
pls
How can i make a comment box like your website?
Here I've shared a blog post about it https://www.codingnepalweb.com/2020/05/copy-text-to-clipboard-button-javascript.html but if you want same to same like this blog post copy codes boxes then I'll send you the codes..Please contact me through the contact page.
How can i make a copyable text box in a post like your website?