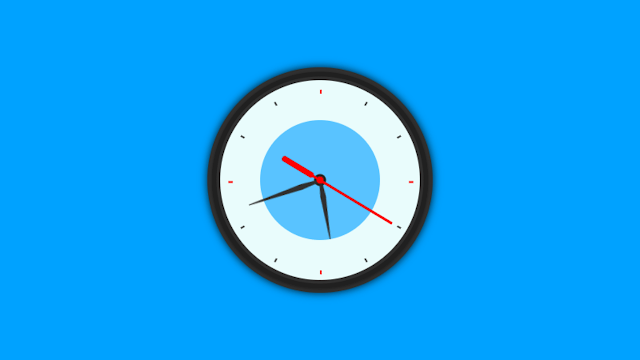
You can tell JavaScript is the core of any web page, in other words, without JavaScript, a web page just is a dead body. We can create so many basic to high-level programs only using JavaScript (without any javascript libraries).
As you can in the image, this is an Analog Clock using HTML CSS & Javascript. This is a real-time clock, not a dummy. You can find the clock program, but you will get most just CSS stuff. Just a vector of the clock. But I am sharing a live clock, which functions as work in javascript.
If you’re feeling difficulty understanding what I am saying. You can watch a full video tutorial on this program (Build A Working Analog Clock).
Video Tutorial of A Working Analog Clock in Javascript
I hope your doubts are clear now. As you see in the video this is a real-time working clock. This time takes from your PC, not from the server. If you’re feeling difficulty to do code of this clock, Don’t worry you can easily get the source code of this Javascript Clock from the download link which is given below.
If you like this program (Build A Working Analog Clock) and want to get source codes. You can easily get the source codes of this program. To get the source codes you just need to scroll down.
Working Analog Clock using Javascript [Source Codes]
To build this project (Build A Working Analog Clock). First, you need to create two Files one HTML File and another one is CSS File. After creating these files just paste the following codes in your file.
First, create an HTML file with the name of index.html and paste the given codes in your HTML file. Remember, you’ve to create a file with .html extension.
<!DOCTYPE html> <!-- Created By CodingNepal --> <html lang="en" dir="ltr"> <head> <meta charset="utf-8"> <title>Clock</title> <link rel="stylesheet" href="style.css"> <style id="clock-animate"></style> </head> <body> <div class="clock"> <div class="center-nut"></div> <div class="center-nut2"></div> <div class="indicator"> <div> </div> <div></div> <div></div> <div></div> <div></div> <div></div> <div></div> <div></div> <div></div> <div></div> <div></div> <div></div> </div> <div class="sec-hand"></div> <div class="min-hand"></div> <div class="hr-hand"></div> </div> <script> (function() { var time = new Date(), //we get this time from our pc time not from server second = time.getSeconds() / 60 * 360, minute = time.getMinutes() / 60 * 360 + time.getSeconds() / 60 *6, hour = time.getHours() / 12 * 360 + time.getMinutes() /60 * 30, animation = [ "@keyframes sec-hand{from{transform: rotate(" + second + "deg);}to{transform: rotate(" + (second + 360) + "deg);}}", "@keyframes min-hand{from{transform: rotate(" + minute + "deg);}to{transform: rotate(" + (minute + 360) + "deg);}}", "@keyframes hr-hand{from{transform: rotate(" + hour + "deg);}to{transform: rotate(" + (hour + 360) + "deg);}}" ].join(""); document.querySelector("#clock-animate").innerHTML = animation; })(); </script> </body> </html>
Second, create a CSS file with the name of style.css and paste the given codes in your CSS file. Remember, you’ve to create a file with .css extension.
*{ margin: 0; padding: 0; } body{ display: flex; align-items: center; justify-content: center; min-height: 100vh; background: #00a2ff; } .clock{ position: relative; display: flex; justify-content: center; align-items: center; width: 250px; height: 250px; background: #e9fcfc; border-radius: 50%; border: 16px solid #303030; box-shadow: 0px 0px 10px 1px rgba(0,0,0,0.8); } .clock:before{ position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); content: ''; width: 259px; height: 259px; border: 6px solid #202020; border-radius: 50%; box-shadow: 0 1px 4px rgba(0, 0, 0, 0.5); } .clock:after{ position: absolute; content: ''; height: 150px; width: 150px; border-radius: 50%; background: #59c3ff; z-index: 1; } .center-nut,.center-nut2{ position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); border-radius: 50%; } .center-nut{ height: 15px; width: 15px; background: #262626; z-index: 2; } .center-nut2{ width: 9px; height: 9px; background: red; z-index: 6; } .indicator div{ position: absolute; width: 2px; height: 5px; background: #404040; } .indicator div:nth-child(1) { transform: rotate(30deg) translateY(-113px); } .indicator div:nth-child(2) { transform: rotate(60deg) translateY(-113px); } .indicator div:nth-child(3) { transform: rotate(90deg) translateY(-113px); background: red; } .indicator div:nth-child(4) { transform: rotate(120deg) translateY(-113px); } .indicator div:nth-child(5) { transform: rotate(150deg) translateY(-113px); } .indicator div:nth-child(6) { transform: rotate(180deg) translateY(-113px); background: red; } .indicator div:nth-child(7) { transform: rotate(210deg) translateY(-113px); } .indicator div:nth-child(8) { transform: rotate(240deg) translateY(-113px); } .indicator div:nth-child(9) { transform: rotate(270deg) translateY(-113px); background: red; } .indicator div:nth-child(10) { transform: rotate(300deg) translateY(-113px); } .indicator div:nth-child(11) { transform: rotate(330deg) translateY(-113px); } .indicator div:nth-child(12) { transform: rotate(360deg) translateY(-113px); background: red; } .sec-hand{ position: absolute; height: 1px; width: 1px; z-index: 5; animation: sec-hand 60s linear infinite; } .sec-hand:before{ position: absolute; content: ''; height: 130px; width: 3px; left: -1px; top: -25px; background: red; border-radius: 3px; } .sec-hand:after{ position: absolute; content: ''; height: 45px; width: 7px; left: -3px; top: -55px; background: red; border-radius: 3px; } .min-hand{ position: absolute; height: 1px; width: 1px; z-index: 4; animation: min-hand 3600s linear infinite; } .min-hand:before{ position: absolute; content: ''; border-left: 3px solid transparent; border-right: 3px solid transparent; border-bottom: 70px solid #303030; left: -3px; top: -95px; width: 1px; height: 1px; } .min-hand:after{ position: absolute; content: ''; border-left: 2px solid transparent; border-right: 2px solid transparent; border-top: 25px solid #303030; left: -3px; top: -25px; width: 3px; height: 1px; } .hr-hand{ position: absolute; height: 1px; width: 1px; z-index: 3; animation: hr-hand 43200s linear infinite; } .hr-hand:before{ position: absolute; content: ''; border-left: 3px solid transparent; border-right: 3px solid transparent; border-bottom: 55px solid #303030; left: -3px; top: -75px; width: 1px; height: 1px; } .hr-hand:after{ position: absolute; content: ''; border-left: 2px solid transparent; border-right: 2px solid transparent; border-top: 20px solid #303030; left: -3px; top: -20px; width: 3px; height: 1px; }
That’s all, now you’ve successfully Build A Working Analog Clock using HTML CSS & Javascript. If your code does not work or you’ve faced any error/problem then please comment down or contact us from the contact page.
Which syntax highlighter do you use
I use Enlighter – Customizable Syntax Highlighter